Update: You can also make this work with real PS3 controllers
In a previous post, we did some warmups — playing MSX games using a fake PS3 controller. In this post, we’ll be using GP2040-CE to control a Nintendo Switch. (No analog stick support implemented at the moment, but it wouldn’t be hard.) At the time of writing, most of the code to do this is already there! And I’m sure there will be support for USB-HID controllers in no time, so this post will probably be outdated soon.
Update: Analog is implemented too, and the diff below has been updated.
Anyway, you just need to put GP2040-CE on your Pico and get into the web configuration. In add-ons, you enable keyboard support, and then set up the “Keyboard Host Configuration”, which looks like this:
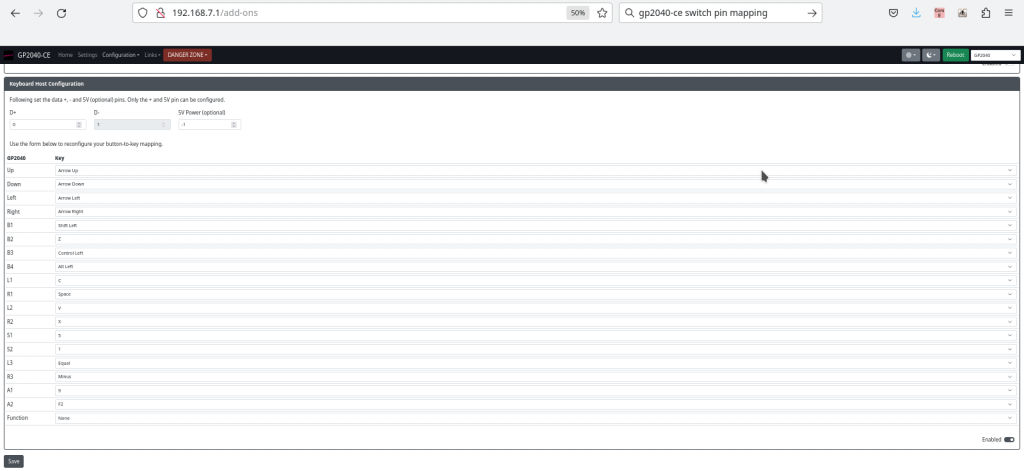
Then you can connect a generic USB keyboard to the Raspberry Pi Pico, and connect the Pico to the Nintendo Switch. (For electrical reasons, I do not recommend setting a pin for 5V power here, and just putting the host USB +5 on the VBUS pin of the Pico.)
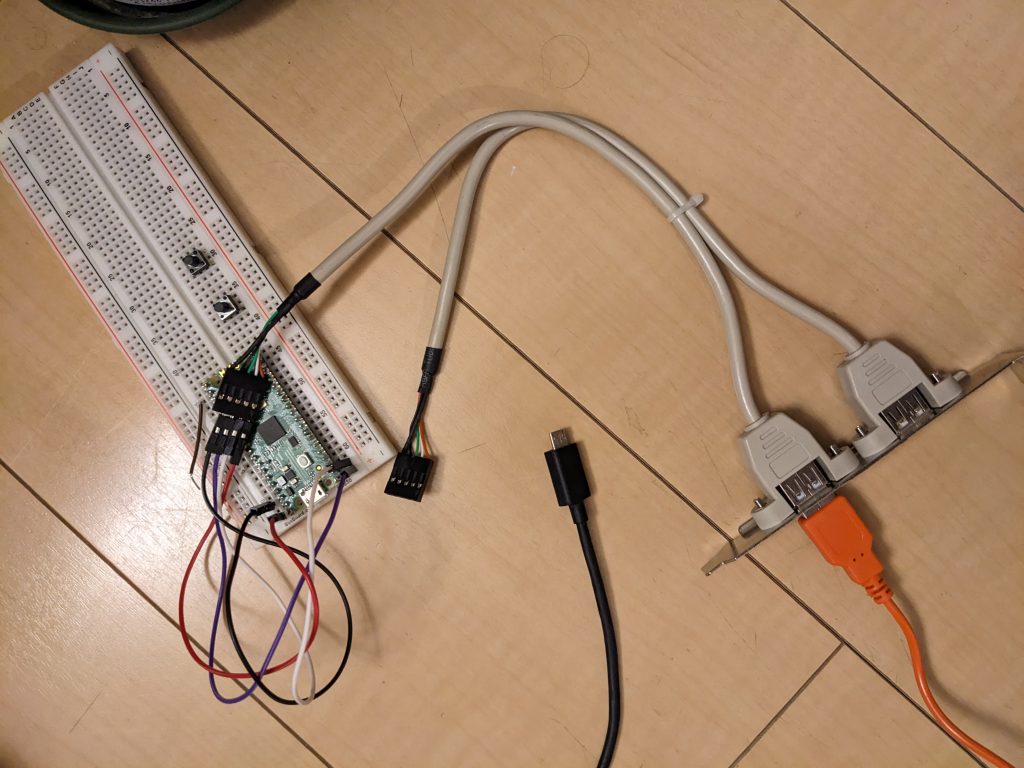
If everything works and you can control Sonic using your keyboard, great, you can move on to the next step! If it didn’t work, it probably won’t magically get better from here on out, so make sure to check those connections. The green/white wires can be D+/D- or D-/D+!
Now we’ll perform a small modification to the existing code. I’m basing my work on commit 961c49d5b969ee749ae17bd4cbb2f0bad2380e71. Beware, this may or may not work with your controller. I’d recommend taking a look at the above-mentioned previous post where we modify a Pico-PIO-USB example and to check if your controller behaves the same way. I have only two controllers to test with, and I only tested with one! Anyway, here’s the diff:
diff --git a/headers/addons/keyboard_host.h b/headers/addons/keyboard_host.h
index af9c61b..74fb628 100644
--- a/headers/addons/keyboard_host.h
+++ b/headers/addons/keyboard_host.h
@@ -53,6 +53,7 @@ private:
bool _keyboard_host_enabled;
uint8_t getKeycodeFromModifier(uint8_t modifier);
void process_kbd_report(uint8_t dev_addr, hid_keyboard_report_t const *report);
+ void process_usb_gamepad_report(uint8_t dev_addr, const uint8_t *report);
GamepadState _keyboard_host_state;
KeyboardButtonMapping _keyboard_host_mapDpadUp;
KeyboardButtonMapping _keyboard_host_mapDpadDown;
@@ -74,4 +75,4 @@ private:
KeyboardButtonMapping _keyboard_host_mapButtonA2;
};
-#endif // _KeyboardHost_H_
\ No newline at end of file
+#endif // _KeyboardHost_H_
diff --git a/src/addons/keyboard_host.cpp b/src/addons/keyboard_host.cpp
index a5294e9..8f59f4a 100644
--- a/src/addons/keyboard_host.cpp
+++ b/src/addons/keyboard_host.cpp
@@ -63,12 +63,15 @@ void KeyboardHostAddon::setup() {
void KeyboardHostAddon::preprocess() {
Gamepad *gamepad = Storage::getInstance().GetGamepad();
+ gamepad->setDpadMode(DpadMode::DPAD_MODE_DIGITAL);
+ gamepad->hasLeftAnalogStick = true;
+ gamepad->hasRightAnalogStick = true;
gamepad->state.dpad |= _keyboard_host_state.dpad;
gamepad->state.buttons |= _keyboard_host_state.buttons;
- gamepad->state.lx |= _keyboard_host_state.lx;
- gamepad->state.ly |= _keyboard_host_state.ly;
- gamepad->state.rx |= _keyboard_host_state.rx;
- gamepad->state.ry |= _keyboard_host_state.ry;
+ gamepad->state.lx = _keyboard_host_state.lx;
+ gamepad->state.ly = _keyboard_host_state.ly;
+ gamepad->state.rx = _keyboard_host_state.rx;
+ gamepad->state.ry = _keyboard_host_state.ry;
gamepad->state.lt |= _keyboard_host_state.lt;
gamepad->state.rt |= _keyboard_host_state.rt;
}
@@ -89,10 +92,11 @@ void KeyboardHostAddon::report_received(uint8_t dev_addr, uint8_t instance, uint
uint8_t const itf_protocol = tuh_hid_interface_protocol(dev_addr, instance);
// tuh_hid_report_received_cb() will be invoked when report is available
- if (itf_protocol != HID_ITF_PROTOCOL_KEYBOARD)
- return;
-
- process_kbd_report(dev_addr, (hid_keyboard_report_t const*) report );
+ if (itf_protocol == HID_ITF_PROTOCOL_KEYBOARD) {
+ process_kbd_report(dev_addr, (hid_keyboard_report_t const*) report );
+ } else {
+ process_usb_gamepad_report(dev_addr, report);
+ }
}
uint8_t KeyboardHostAddon::getKeycodeFromModifier(uint8_t modifier) {
@@ -161,4 +165,96 @@ void KeyboardHostAddon::process_kbd_report(uint8_t dev_addr, hid_keyboard_report
_keyboard_host_state.rt = 0;
}
}
-}
\ No newline at end of file
+}
+
+// 0x1: L2
+// 0x2: R2
+// 0x4: L1
+// 0x8: R1
+// 0x10: Triangle
+// 0x20: Circle
+// 0x40: X
+// 0x80: Square?
+// 0x100: ?
+// 0x200: ?
+// 0x400: R3
+// 0x800: Start
+// 0x1000: Up
+// 0x2000: Right
+// 0x4000: Down?
+// 0x8000: Left?
+
+#define BUTTON_L2 0x1
+#define BUTTON_R2 0x2
+#define BUTTON_L1 0x4
+#define BUTTON_R1 0x8
+#define BUTTON_SQUARE 0x10
+#define BUTTON_CROSS 0x20
+#define BUTTON_CIRCLE 0x40
+#define BUTTON_TRIANGLE 0x80
+
+#define BUTTON_SELECT 0x100 // ?
+#define BUTTON_L3 0x200 // ?
+#define BUTTON_R3 0x400 // ?
+
+#define BUTTON_START 0x800
+#define BUTTON_UP 0x1000
+#define BUTTON_RIGHT 0x2000
+#define BUTTON_DOWN 0x4000
+#define BUTTON_LEFT 0x8000
+
+void KeyboardHostAddon::process_usb_gamepad_report(uint8_t dev_addr, const uint8_t *report)
+{
+ _keyboard_host_state.dpad = 0;
+ _keyboard_host_state.buttons = 0;
+ _keyboard_host_state.lx = GAMEPAD_JOYSTICK_MID;
+ _keyboard_host_state.ly = GAMEPAD_JOYSTICK_MID;
+ _keyboard_host_state.rx = GAMEPAD_JOYSTICK_MID;
+ _keyboard_host_state.ry = GAMEPAD_JOYSTICK_MID;
+ _keyboard_host_state.lt = 0;
+ _keyboard_host_state.rt = 0;
+
+ uint16_t button_state = report[2] << 8 | report[3];
+ uint8_t left_analog_x = report[6];
+ uint8_t left_analog_y = report[7];
+ uint8_t right_analog_x = report[8];
+ uint8_t right_analog_y = report[9];
+
+ const GamepadOptions& gamepadOptions = Storage::getInstance().getGamepadOptions();
+
+ _keyboard_host_state.dpad |=
+ ((button_state & BUTTON_UP) ? (gamepadOptions.invertYAxis ? _keyboard_host_mapDpadDown.buttonMask : _keyboard_host_mapDpadUp.buttonMask) : _keyboard_host_state.dpad)
+ | ((button_state & BUTTON_DOWN) ? (gamepadOptions.invertYAxis ? _keyboard_host_mapDpadUp.buttonMask : _keyboard_host_mapDpadDown.buttonMask) : _keyboard_host_state.dpad)
+ | ((button_state & BUTTON_LEFT) ? _keyboard_host_mapDpadLeft.buttonMask : _keyboard_host_state.dpad)
+ | ((button_state & BUTTON_RIGHT) ? _keyboard_host_mapDpadRight.buttonMask : _keyboard_host_state.dpad)
+ ;
+
+ _keyboard_host_state.buttons |=
+ ((button_state & BUTTON_CROSS) ? _keyboard_host_mapButtonB1.buttonMask : _keyboard_host_state.buttons)
+ | ((button_state & BUTTON_CIRCLE) ? _keyboard_host_mapButtonB2.buttonMask : _keyboard_host_state.buttons)
+ | ((button_state & BUTTON_SQUARE) ? _keyboard_host_mapButtonB3.buttonMask : _keyboard_host_state.buttons)
+ | ((button_state & BUTTON_TRIANGLE) ? _keyboard_host_mapButtonB4.buttonMask : _keyboard_host_state.buttons)
+ | ((button_state & BUTTON_L1) ? _keyboard_host_mapButtonL1.buttonMask : _keyboard_host_state.buttons)
+ | ((button_state & BUTTON_R1) ? _keyboard_host_mapButtonR1.buttonMask : _keyboard_host_state.buttons)
+ | ((button_state & BUTTON_L2) ? _keyboard_host_mapButtonL2.buttonMask : _keyboard_host_state.buttons)
+ | ((button_state & BUTTON_R2) ? _keyboard_host_mapButtonR2.buttonMask : _keyboard_host_state.buttons)
+ | ((button_state & BUTTON_SELECT) ? _keyboard_host_mapButtonS1.buttonMask : _keyboard_host_state.buttons)
+ | ((button_state & BUTTON_START) ? _keyboard_host_mapButtonS2.buttonMask : _keyboard_host_state.buttons)
+ | ((button_state & BUTTON_L3) ? _keyboard_host_mapButtonL3.buttonMask : _keyboard_host_state.buttons)
+ | ((button_state & BUTTON_R3) ? _keyboard_host_mapButtonR3.buttonMask : _keyboard_host_state.buttons)
+ ;
+
+ /*
+ * #define GAMEPAD_JOYSTICK_MIN 0
+ * #define GAMEPAD_JOYSTICK_MID 0x7FFF
+ * #define GAMEPAD_JOYSTICK_MAX 0xFFFF
+ * lx, ly, rx, ry are 16-bit values, but our joystick produces 8-bit values
+ * our joystick's middle is at 0x80, so it would probably be slightly better to adjust that.
+ */
+ _keyboard_host_state.lx = (left_analog_x == 0x80) ? GAMEPAD_JOYSTICK_MID : (left_analog_x << 8 | left_analog_x);
+ _keyboard_host_state.ly = (left_analog_y == 0x80) ? GAMEPAD_JOYSTICK_MID : (left_analog_y << 8 | left_analog_y);
+ _keyboard_host_state.rx = (right_analog_x == 0x80) ? GAMEPAD_JOYSTICK_MID : (right_analog_x << 8 | right_analog_x);
+ _keyboard_host_state.ry = (right_analog_y == 0x80) ? GAMEPAD_JOYSTICK_MID : (right_analog_y << 8 | right_analog_y);
+ _keyboard_host_state.lt = 0;
+ _keyboard_host_state.rt = 0;
+}
Good luck. Miraculously, everything worked perfectly for me. The keyboard worked immediately, the above code modification worked immediately without having to do any debugging, and I’ve gotta say, my fake PS3 controller feels quite okay! (Note that you will have to press the PlayStation button after connecting your PS3 controller.)